With the aid of examples, we will learn the fundamentals of C++ type conversion in this tutorial.
C++ Type Conversion
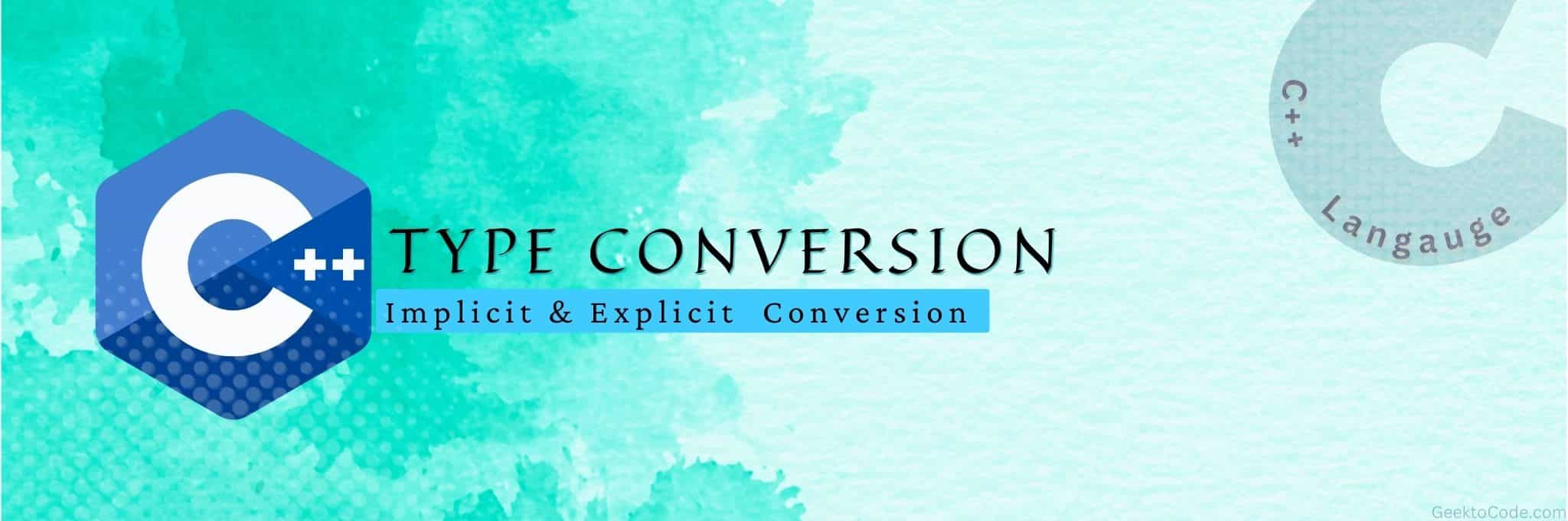
We can change data from one type to another using C++. We call this type conversion.
In C++, there are two different types of type conversion:
- Implicit Conversion
- Explicit Conversion (also known as Type Casting)
Implicit Conversion:
- It is also referred to as “automatic type conversion.”
- Without any external trigger from the user, carried out by the compiler on its own.
- This typically happens when there are multiple data types in an expression. To prevent data loss, type conversion (type promotion) occurs in such circumstances.
bool -> char -> short int -> int -> unsigned int -> long -> unsigned -> long long -> float -> double -> long double
- All variable data types have been upgraded to the variable’s data type with the largest data type.
- When signed is implicitly converted to unsigned, information may be lost during implicit conversions, signs may be lost, and overflow may occur (when long long is implicitly converted to float).
EXAMPLE:
// An example of implicit conversion
#include <iostream>
using namespace std;
int main()
{
// Program by GeektoCode
int x = 55; // integer x
char y = 'a'; // character c
// y implicitly converted to int. ASCII
// value of 'a' is 97
x = x + y;
// x is implicitly converted to float
float z = x + 1.0;
cout << "x = " << x << endl
<< "y = " << y << endl
<< "z = " << z << endl;
return 0;
}
Output:
x = 152
y = a
z = 153
Loss of Data During Conversion
As we can see from the example above, data loss can occur when converting between different data types. This occurs when larger-type data is transformed into smaller-type data.
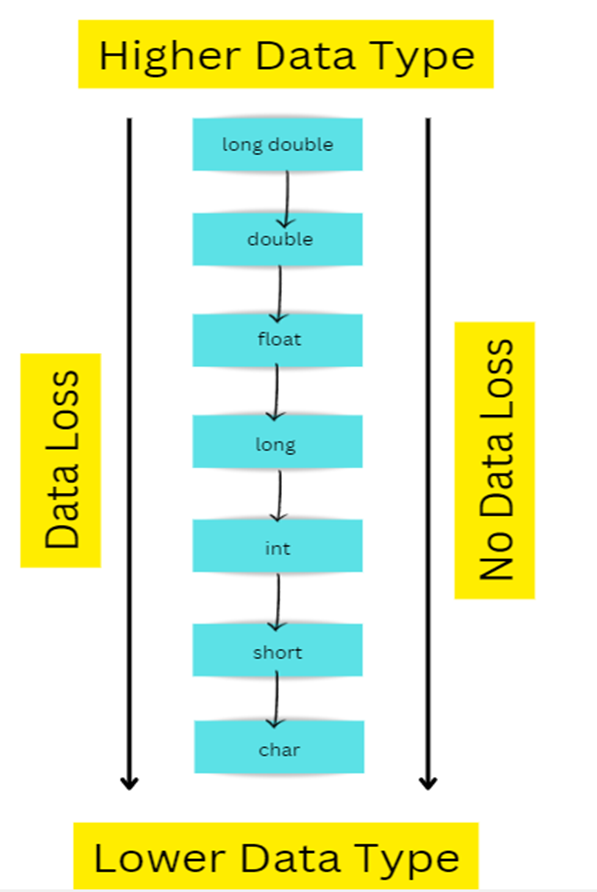
Explicit Type Conversion:
It is a user-defined process that is also known as type casting. The user can typecast the result, in this case, to convert it to a specific data type.
It can be done in C++ in one of two ways:
- Converting by assignment
- Conversion using Cast operator
Converting by assignment:
In order to achieve this, the required type must be explicitly defined before the expression in parenthesis. This might also be regarded as forced casting.
Syntax:
(type) expression
where type denotes the final conversion of the result’s data.
EXAMPLE:
// explicit type casting
#include <iostream>
using namespace std;
int main()
{
//Program by GeektoCode
double x = 8.2;
// Explicit conversion from double to int
int sum = (int)x + 7;
cout << "Sum = " << sum;
return 0;
}
Output:
Sum = 15
Using the Cast operator to convert data:
The Cast operator is a unary operator that compels the conversion of one data type into another.
Four casting types are supported by C++:
- Static Cast
- Dynamic Cast
- Const Cast
- Reinterpret Cast
EXAMPLE:
#include <iostream>
using namespace std;
int main()
{
//Program by GeektoCode
float f = 10.4;
// using cast operator
int b = static_cast<int>(f);
cout << b;
}
Output:
Sum = 10
Explicit conversion occurs when a user manually converts data from one type to another. Type casting is another name for this type of conversion.
In C++, there are primarily three ways to use explicit conversion. As follows:
- casting in the C-style (referred to as cast notation)
- Function notation also referred to as type casting in the old C++ fashion
- Operators for converting types